Extensions
Extension A: Invite a Guest
- Invite a guest speaker to talk about Indigenous music - Drummer, Jigger, Powwow Dancer, Throat Singer, Indigenous Musician, or Dancer.
- Create a list of questions in advance to ask the guest speaker.
Extension B: Create a Function with Variables
The functions we created are ‘hard-coded’ which means every time you call the function they do EXACTLY the same thing. Using variables we can pass in values to change what happens when the function is called. Variables passed to functions are called parameters.
Follow the instructions below or in your student coding workbook to create a function with variables
Related Video tutorials:
- The chorus of a song often repeats, so try repeating the chorus in your song by calling the chorus function twice in the # music section
# music section
intro()
chorus()
chorus()
- Run your code and play your song.
- Does the chorus play twice?
The code runs and the song plays, but you only hear the chorus once.
When you select RUN a warning message appears at the bottom of your screen that gives you a hint
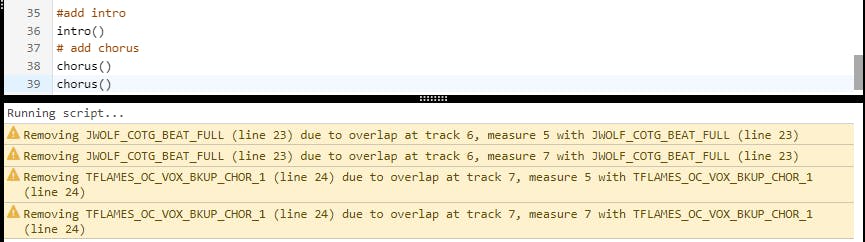
When you call chorus() the second time it executes EXACTLY the same commands again. That means it tried to add the same sounds on the same tracks and measures twice!
- Add variables called start and end to your function definition by listing them in the parentheses beside the function name.
def chorus(start,end):
- When you call fitMedia() in the chorus(start, end) function, use the variable start and end instead of ‘hard-coding’ the values for the start and end measures
def chorus(start,end):
fitMedia(drumchorus,6,start,end)
fitMedia(vocalchorus,7,start,end)
- Each time you call chorus() pass in the numbers to use for the start and end measures. To play the chorus at different times in the song, just pass in different start and end values. The code below would play the chorus three times: once from measures 5 to 9; a second time from measure 15 to 19; a third time from measures 24 to 30
# music section
intro()
chorus(5,9)
chorus(15,19)
chorus(24,30)
- Run your code and look at the DAW. Scroll over to see the extra chorus. Play the song and let it play until the end, do you hear the chorus starting again after a pause.
The finished code would look similar to the following
# description:
from earsketch import *
setTempo(120)
# SOUNDBANK
#drums
drums = SAMIAN_PEUP_BEAT_FULL
#bass
bass = TFLAMES_OC_BASS_CHOR
#flute
flute = SAMIAN_PEUP_THEME_FLUTE
#strings
strings = SAMIAN_PEUP_THEME_STRINGS_3
#vocals
vocal1 = JWOLF_COTG_VOX_MISC_SHOUT
#Chorus sounds
drumchorus = JWOLF_COTG_BEAT_FULL
vocalchorus = TFLAMES_OC_VOX_BKUP_CHOR_1
# Define chorus function pass in the start and end measure values
def chorus(start, end):
fitMedia(drumchorus,6, start, end)
fitMedia(vocalchorus,7, start, end)
# Define intro function
def intro():
fitMedia(drums, 1,1,5)
fitMedia(bass,2,1,5)
fitMedia(flute,3,1,3)
fitMedia(strings,4,1,3)
fitMedia(vocal1,5,3,5)
#add intro
intro()
# add chorus pass in start and end measure values
chorus(5,9)
chorus(15,19)
chorus(24,30)
Mastering custom functions with variables is the most advanced coding concept in Your Voice is Power, so it may take you a little while to get the hang of it. It’s okay to come back to this later.
Extension C: Create a Custom Functions with Variables and Staggered Start and End Measures
What if we don’t want the sounds in our chorus to start and end at the same time? For example, what if for our chorus we want the drum to play for the entire chorus, but we want to wait a measure before the vocals start.
If we hardcoded the fitMedia calls we could specify a different start measure for the vocals, we can specify a start measure of 5 for the drums and a start measure of 6 for the vocals so they start shortly after the drums.
fitMedia(drumchorus,6, 5, 9)
fitMedia(vocalchorus,7, 6, 9)
In our function we use variables to specify the start measure
def chorus(start, end):
fitMedia(drumchorus,6, start, end)
fitMedia(vocalchorus,7, start, end)
If you want to have one sound start on a different measure, you can use math operators on the start variable when you use it in the function.
def chorus(start, end):
fitMedia(drumchorus,6, start, end)
fitMedia(vocalchorus,7, start+1, end)
Do the math on start measures
In your Coding Activity Workbook, try to figure out the values fitMedia will use for the start measure for each sound in the above code based on different values passed into the chorus function
What will be the start measure used for drumchorus? and vocalchorus?
If we call the chorus function using
chorus(5,9)
chorus(15,19)
chorus(24,30)
If you want to have one sound end on a different measure, you can use math operators on the end variables passed to the function
def chorus(start, end):
fitMedia(drumchorus,6, start, end)
fitMedia(vocalchorus,7, start, end-1)
Do the math on end measures
What will be the end measure used for drumchorus? and vocalchorus?
If we call the chorus function using
chorus(5,9)
chorus(15,19)
chorus(24,30)
Try it in your code
Modify your chorus function so one of the sounds starts or ends on a different measure from the other sounds in the chorus.
The finished code will look similar to the following:
# description:
from earsketch import *
setTempo(120)
# SOUNDBANK
#drums
drums = SAMIAN_PEUP_BEAT_FULL
#bass
bass = TFLAMES_OC_BASS_CHOR
#flute
flute = SAMIAN_PEUP_THEME_FLUTE
#strings
strings = SAMIAN_PEUP_THEME_STRINGS_3
#vocals
vocal1 = JWOLF_COTG_VOX_MISC_SHOUT
#Chorus sounds
drumchorus = JWOLF_COTG_BEAT_FULL
vocalchorus = TFLAMES_OC_VOX_BKUP_CHOR_1
# Define chorus function
# pass in the start and end measure values
# start the vocals 1 measure after the drums start
# end the vocals 1 measure before the drums end
def chorus(start, end):
fitMedia(drumchorus,6, start, end)
fitMedia(vocalchorus,7, start+1, end-1)
# Define intro function
def intro():
fitMedia(drums, 1,1,5)
fitMedia(bass,2,1,5)
fitMedia(flute,3,1,3)
fitMedia(strings,4,1,3)
fitMedia(vocal1,5,3,5)
#add intro
intro()
# add chorus pass in start and end measure values
chorus(5,9)
chorus(15,19)
chorus(24,30)
Extension D: Participate in a Sharing Circle (20 minutes)
Take turns passing around the talking object.
- Do you know any Indigenous role models?
- What do they do?
About the Sharing Circle:
- Whoever has the talking object is The Speaker.
- The Speaker may speak for as long as they need to, with respect for the time of others.
- Silence is an acceptable response to the question.
- If a student does not feel comfortable sharing, “I pass” is an acceptable answer.
- Speaking in a Sharing Circle is voluntary.
- Everyone without the talking object are listeners.
- Listeners will listen with respect and give support to the speaker.
- Listen in the way you expect others to hear you.
- What is said in the circle stays in the circle - never repeat anything that is said within the circle, unless you have permission from the speaker.
Optional:
Invite an Elder, Traditional Knowledge Keeper, or Indigenous Storyteller to the classroom to talk about the importance of storytelling and/or Indigenous knowledge.